Getting Started
We will describe how to make and run your first Streamlabs OBS app in 5 minutes!
Setting up
Once you are an approved developer, any build of Streamlabs Desktop should work for local app development. However, we recommend you run on our Preview build to always be working against our latest changes. You can download the latest preview build for Windows here, and macOS here
Create your application
Open the Streamlabs Platform Site and press the Create App
button. Your application will be immediately created.
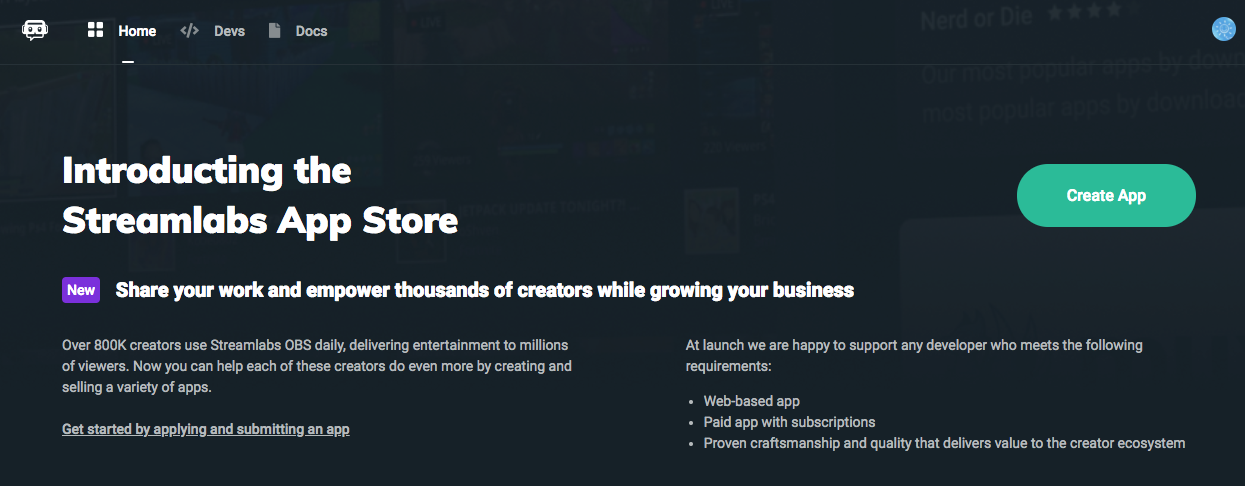
Click Create App
to create a new application
You can see that you will have to provide some details about your application, but you can do this later before going live. You can skip this step for now.
Start your project
First, you should create a new folder on your PC that will contain your project source. Each Streamlabs Platform application should contain a manifest.json
file - this describes your application structure to Streamlabs Desktop.
The minimal folder structure for your first Streamlabs Platform app should look like below:
|-- my-first-app
|-- index.html
|-- manifest.json
Where index.html
contains your application logic.
Include the Platform API script
To use our SDK and get access to the Streamlabs Platform API, you should include our helper library in the <script>
section of your HTML
<html>
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Page Title</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<script src="https://cdn.streamlabs.com/slobs-platform/lib/streamlabs-platform.min.js"></script>
</head>
....
Code your application
You can use whatever JavaScript frameworks or libraries that you want or even just use pure JS. Don't forget to initialize the SDK to receive authentication details!
An introduction and complete reference for the Platform API can be found here: Platform API
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<script src="https://cdn.streamlabs.com/slobs-platform/lib/streamlabs-platform.min.js"></script>
<title>First Widget</title>
</head>
<body>
<div id="content">Hello, World!</div>
<script>
const colors = ['red', 'blue', 'green', 'orange', 'purple', 'black', 'gold'];
const streamlabs = window.Streamlabs;
streamlabs.init({ receiveEvents: true })
.then(data => {
console.log('auth completed');
});
streamlabs.onFollow(event => {
const follower = event.message[0];
const div = document.getElementById('content');
div.innerHTML = follower.name + ' is now following';
// set random color
const color = colors[Math.floor(Math.random() * colors.length)];
div.setAttribute('style', 'color: ' + color);
});
</script>
</body>
</html>
Add a manifest to your project
The manifest.json
describes your application to Streamlabs Desktop, including:
- the permissions your app needs
- the current version number of your application
- which sources your app exposes (if any)
- what pages your app will inject (if any)
Let's copy the manifest content below for now.
A detailed description of the manifest can be found here
{
"version": "0.0.1",
"name": "Example App",
"permissions": [],
"sources": [
{
"type": "browser_source",
"name": "Example Widget",
"id": "test",
"about": {
"description": "First example widget",
"bullets": []
},
"file": "index.html",
"initialSize": {
"type": "relative",
"width": 1,
"height": 1
}
}
],
"pages": []
}
Copy your App Token
Navigate to the Test App
section on the Streamlabs Platform Site and copy the application token. You will need this token to load your app in test
mode in Streamlabs Desktop.
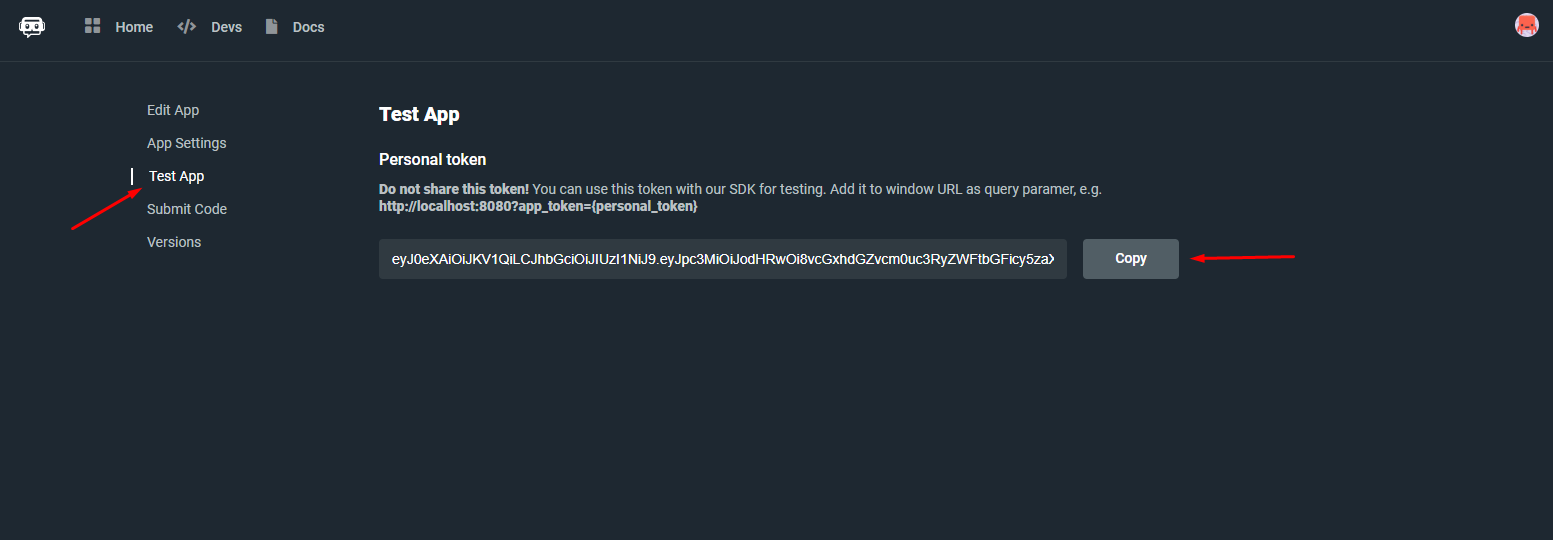
Copy the App Token
Load your application in Streamlabs Desktop
To test application locally with the SDK you should upload your application to Streamlabs Desktop. To do this, open Streamlabs Desktop and click on the settings
icon in right corner:
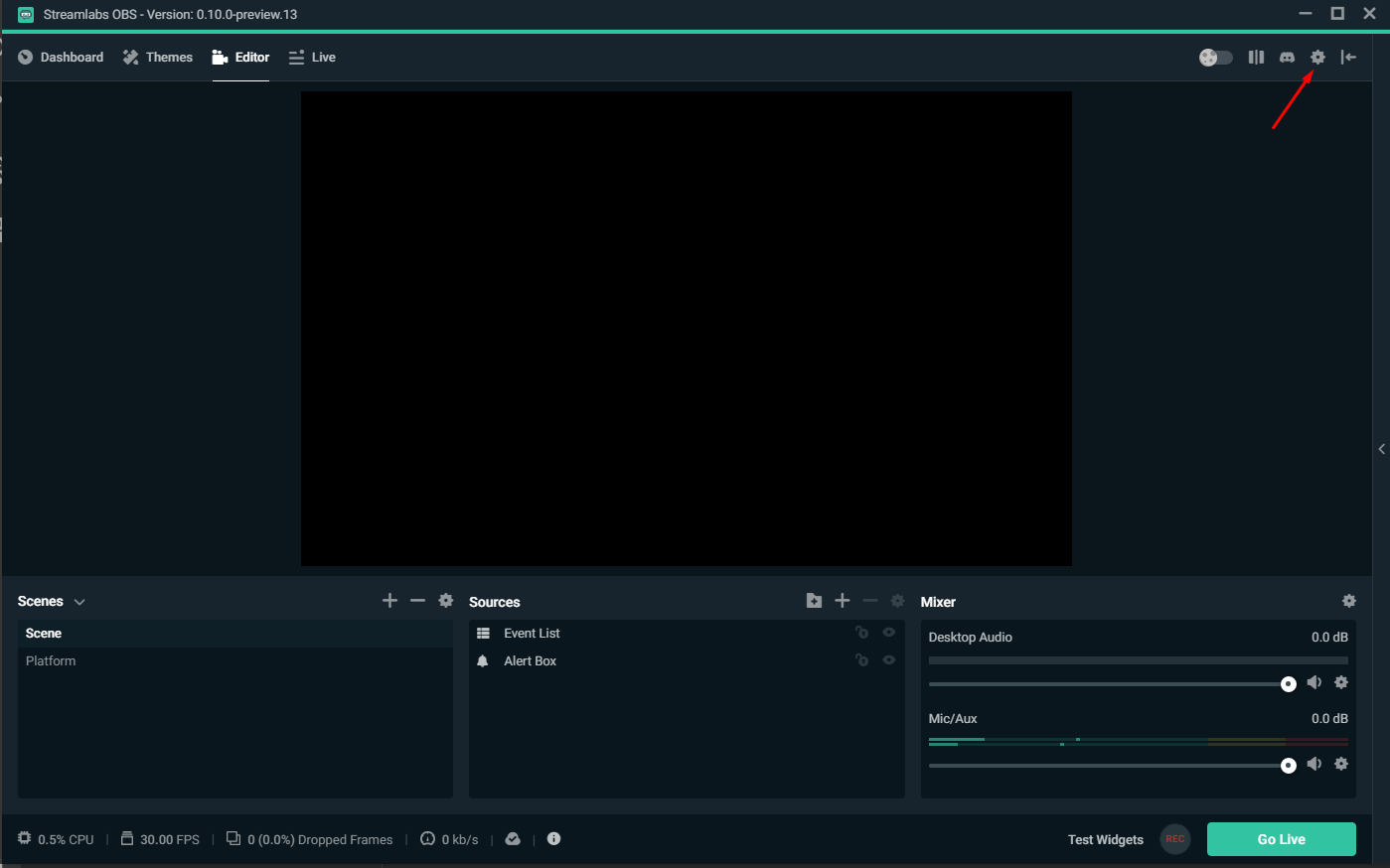
Settings icon location
Then you should navigate to the Developer
section of the settings menu:
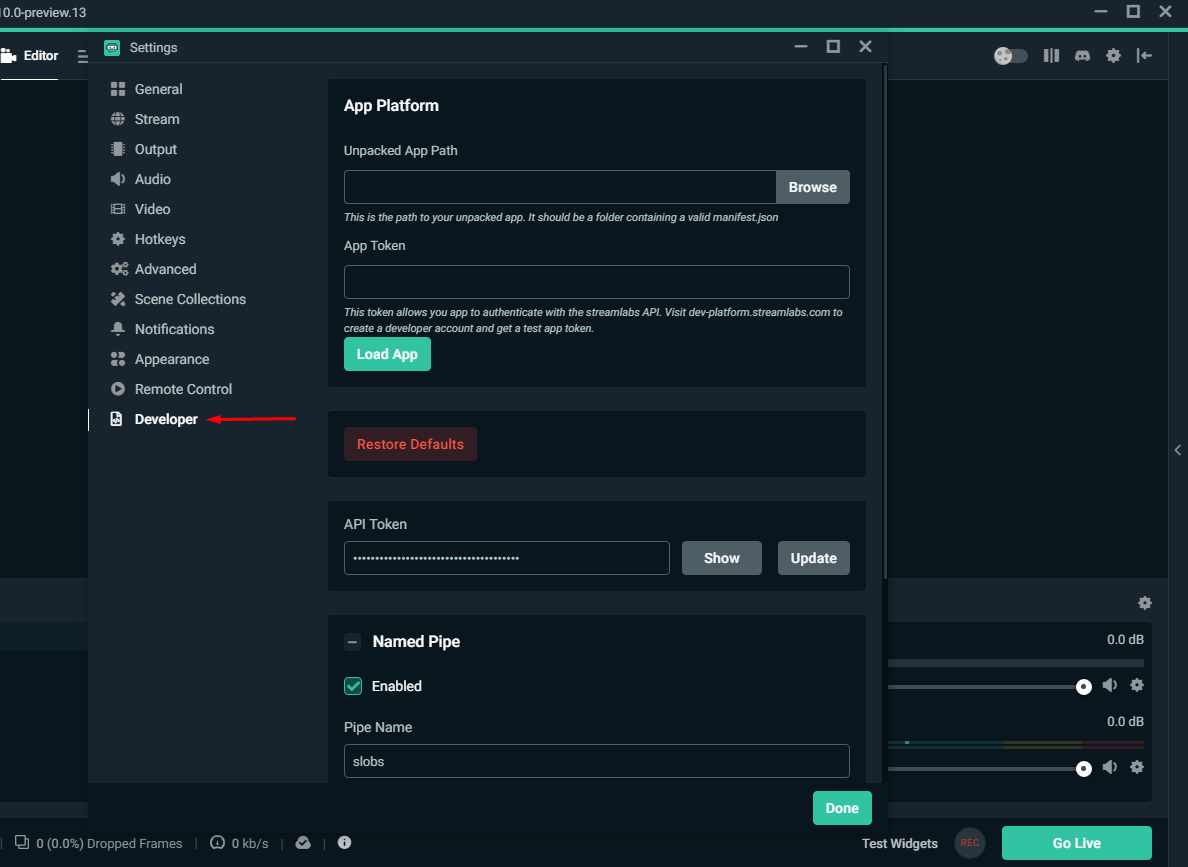
Developer
section in the settings menu
Then you should press the Browse
button and select the folder containing your application and paste the token that you've previously copied into the App Token
input:
Load your application
When you are ready, press the Load App
button.
Add your widget to the current scene
Lastly, you should add your application to the current scene. After this step it will be visible on your stream. To do that, click the plus
icon in the Sources
section of Streamlabs Desktop:
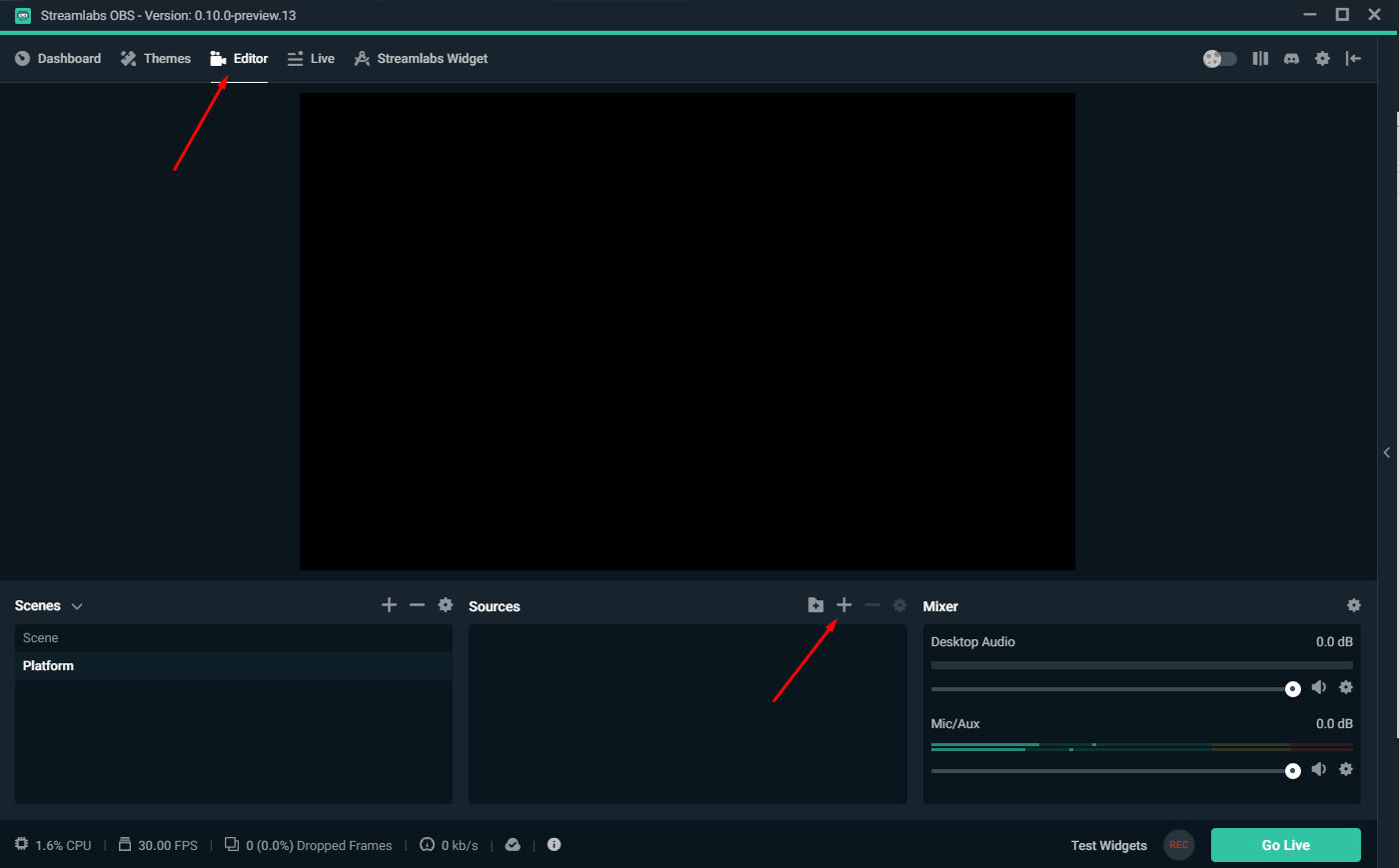
Add a new source
Next, select your application from the Apps
section and follow the instructions:
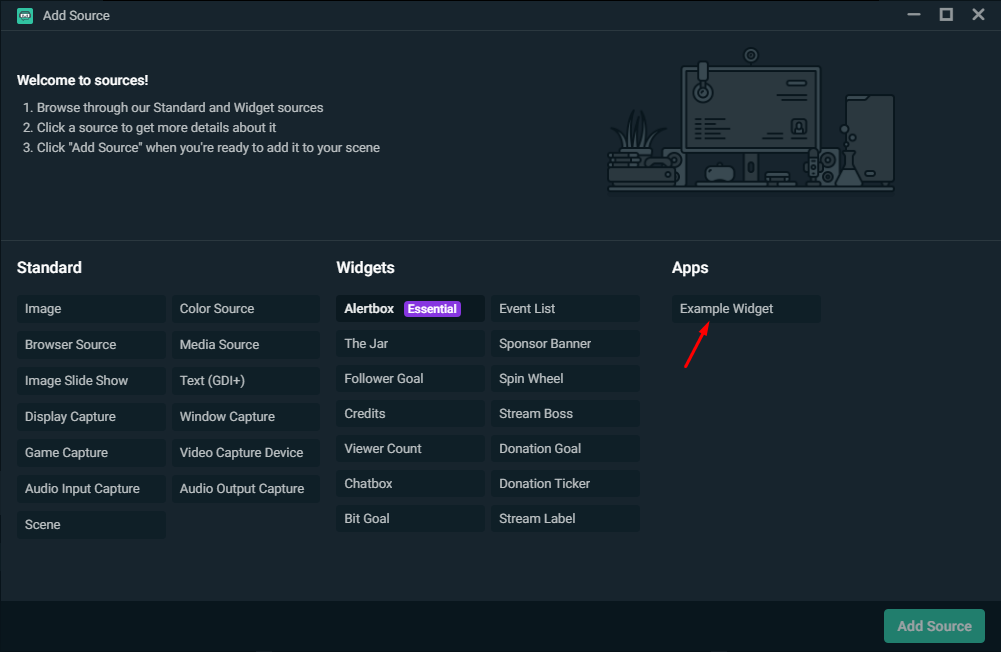
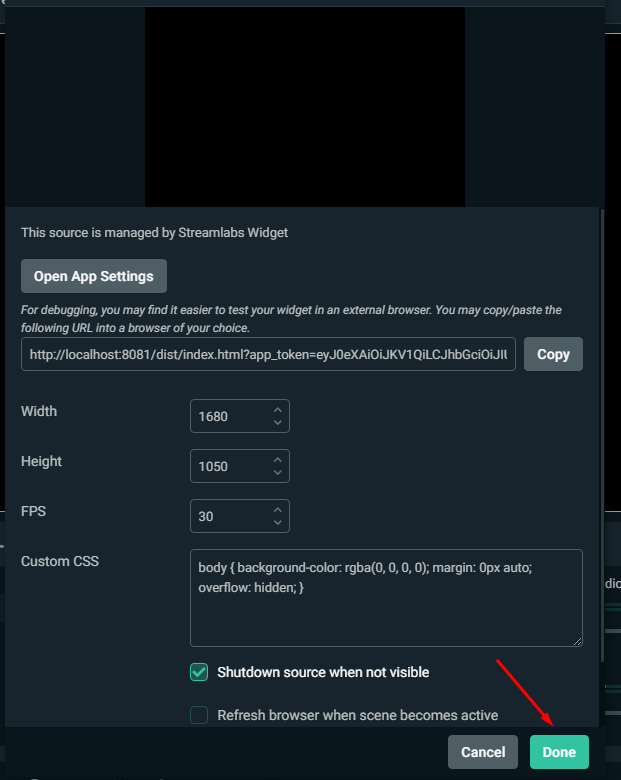
Click Done
on this screen
Thats all: now you have an application that works inside the Streamlabs Desktop. Lets go and test how it works!
Test your application
If you followed all steps of this Getting Started
guide, then your widget should listen for Twitch follow
events from Streamlabs. To test them, press on the Test Widgets
button on the bottom.
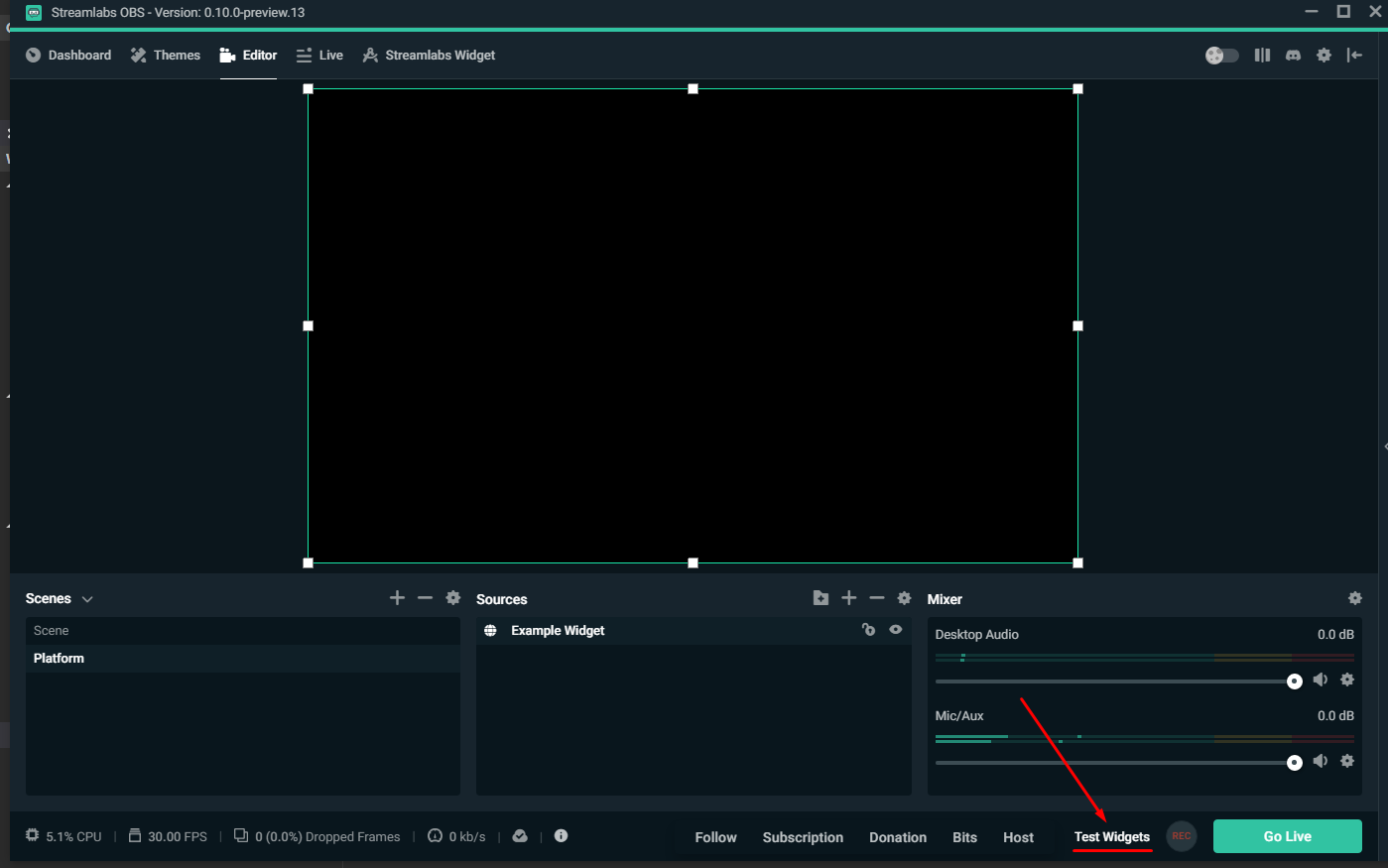
Test Widgets
button
Press the Follow
button and you should receive event in your application.
Updated over 2 years ago