Reference
window.Streamlabs
— Platform API SDK
The JavaScript helper has these functions:
- init
- onError
- onIOConnected
- onFollow
- onSubscription
- onDonation
- onHosting
- onBits
- onRaid
- onMerch
- onSuperchat
- onStreamlabels
- onUnderlyingStreamlabels
- onMessage
- postMessage
- onChatMessage
- onChatEvent
- sendChatMessage
- sendChatRaw
- clear
- userSettings
- userSettings.get
- userSettings.getAll
- userSettings.set
- userSettings.delete
- userSettings.deleteAll
- userSettings.addAssets
- userSettings.removeAssets
- userSettings.getAssets
- streamlabels.getInitialData
- streamlabels.getUnderlyingInitialData
- twitch.connectTwitchChatByName
- twitch.initTwitchChat
- alerts.createAlerts
- alerts.mute
- alerts.unmute
- alerts.repeat
init
init: function(options: Object) Promise
Init SDK and receive profiles information and settings. If required, SDK automatically connects to Twitch Chat and Streamlabs to receive messages and events.
options
is an object with these properties:
Property | Type | Description |
---|---|---|
receiveEvents | bool |
|
twitchChat | bool |
|
init
function returns a Promise, which resolves with an object with these properies:
Property | Type | Description |
---|---|---|
profiles | Object that contains profiles information about application's current user. | |
settings | object | Global settings of your application, that you can provide in |
token | string | Application authentication token |
jwtToken | string | The JWT token signed by your App.Secrect. |
assets | object |
More details here |
init
function rejects a Promise with one of these errors if something goes wrong. You should catch them and handle according to the error type.
Error | Type | Description |
---|---|---|
SubscriptionExpired | subscription-expired | User has expired subscription. |
AuthError | auth | Fired when provided auth token was not valid |
UnknownError | unknown | Fired when something goes wrong on our side |
Profiles object
object
profiles
object contains information about user's linked platforms.
Property | Type | Description |
---|---|---|
primary | string | Which platform is |
twitch | object | Twitch profile data |
youtube | object | YouTube profile data |
mixer | object | Mixer profile data |
object | Facebook profile data |
profiles.twitch
object contains information about twitch
account of your user:
Property | Type | Description |
---|---|---|
twitch_id | string | Twitch channel ID |
display_name | string | Twitch display name (it may be different from |
name | string | Twitch name |
partnered | number | Twitch partner status: |
icon_url | string | Link to user's Twitch avatar icon |
profile_background_color | string | Profile background color on Twitch |
profile_banner_url | string | Link to the profile banner image on Twitch |
profiles.streamlabs
object contains information about streamlabs
account of a user:
Property | Type | Description |
---|---|---|
id | number | Streamlabs user id |
name | string | Username |
prime | boolean | If user have prime subscription |
prime_expires_at | Date (YYYY-MM-DD hh:mm:ss) | Prime subscription expiration date |
profiles.youtube
object contains information about youtube
account of a user:
Property | Type | Description |
---|---|---|
youtube_id | string | YouTube |
name | string | YouTube username |
thumbnail_url | string | Thumbnail URL |
profile_color | string | YouTube background color |
banner_url | string | YouTube background banner URL |
custom_url | string | YouTube custom channel URL suffix. E.g. |
profiles.mixer
object contains information about mixer
account of a user.
Property | Type | Description |
---|---|---|
mixer_id | string | Mixer ID |
name | string | Username |
title | string | Channel title |
profile_color | string | Mixer background color |
banner_url | string | Mixer background image URL |
thumbnail_url | string | Thumbnail URL |
profiles.facebook
object contains information about facebook
account of a user.
Property | Type | Description |
---|---|---|
facebook_id | string | Facebook ID |
name | string | Name |
title | string | Channel title |
thumbnail_url | string | Thumbnail URL |
banner_url | string | Background banner URL |
profile_color | string | Background color |
gender | string | Gender |
For example:
const streamlabs = window.Streamlabs;
// we don't want to receive any events from Streamlabs and dont want to connect to twitch chat
streamlabs.init()
.then(data => {
// twitch profile
const twitchProfile = data.profiles.twitch;
// your application global settings from platform.streamlabs.com
const settings = data.settings;
// e.g. we have set a background color in this settings
const color = settings.color;
// authentication token
const token = data.token;
})
.catch(err => {
// handle auth errors here
console.log(err.type);
if (err.type === 'subscription-expired') {
console.log('subscribe to get access to this application');
}
});
onError
onError: function(onErrorCallback: function) callbackId: string
onErrorCallback is fired if any errors are occurred.
onErrorCallback
is a function with one object argument:
Property | Type | Description |
---|---|---|
type | string | Type of an error. You can differently handle errors based on this type. |
message | string | Text description of an error. |
Error types:
Type | Description |
---|---|
io | Fired when something goes wrong with Streamlabs events connection (source disconnected, network error). SDK reconnects automatically to io server, no action required to establish connection. |
tw-chat | Fired when something goes wrong with Twitch chat connection (source disconnected, network error). SDK reconnects automatically to twitch chat, no action required. |
onIOConnected
Fires when client connects to IO server.
onIOConnected:function(onIOConnectedCallback: function) callbackId:string
onIOConnectedCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event | string | always 'io_connected' |
onFollow
Fires when someone follows a user channel.
onFollow: function(onFollowCallback: function) callbackId: string
onFollowCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always 'follow') |
message | array of objects | Array with details about followers. |
for | string | Platform identifier of event (specifies from which platform did this event come from). Possible values are: |
message
array element object properties:
Property | Type | Description |
---|---|---|
name | string | Twitch name of the follower |
isTest | bool | true if test event is fired from Streamlabs . |
onFollow
event example:
{
"type": "follow",
"event_id": "evt_1efc77fc74c405099e149cc06669ad73",
"for": "twitch_account",
"message": [{
"name": "salmondx",
"isTest": true
}]
}
onSubscription
Fires when someone subscribe to a user channel.
For YouTube
- fired for membership events.
onSubscription: function(onSubscriptionCallback: function) callbackId: string
onSubscriptionCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always 'subscription') |
message | array of objects | Array with details about subscribers. |
for | string | Platform identifier of event (specifies from which platform did this event come from). Possible values are: |
message
array element object properties:
Property | Type | Description |
---|---|---|
name | string | Twitch name of the subscriber |
months | number | Number of months of how long this user is subscribed. |
message | string | Subscriber message |
sub_plan | string | Subscriber subscription plan (?) |
emotes | ? | ? |
isTest | bool | true if test event is fired from Streamlabs . |
onSubscription
event example:
{
"type": "subscription",
"event_id": "evt_9a3697540df48b12a0a4ebb2e99d9e47",
"for": "twitch_account",
"message": [{
"gifter": "sunny", //exists when current sub is a gift sub
"name": "salmondx",
"isTest": true,
"months": 2,
"message": "This is a test",
"emotes": null,
"sub_plan": "1000"
}]
}
onDonation
Fires when someone donate to a user via Streamlabs tipping page.
onDonation: function(onDonationCallback: function) callbackId: string
onDonationCallback
is a function with one argument, an object with these properties:
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always 'donation') |
message | array of objects | Array with details about donations. |
message
array element object properties:
Property | Type | Description |
---|---|---|
from | string | Username from tipping page |
amount | number | Donation amount |
currency | string | Currency code |
formatted_amount | string | Amount and currency combined with currency code replaced with currency symbol if exists. E.g. amount 31 and code USD will become $31.00 . |
message | string | User donation message from tipping page |
isTest | bool | true if test event is fired from Streamlabs . |
name | string | Twitch username of donator if he was logged in to Streamlabs on tipping page |
onDonation
event example:
{
"type": "donation",
"event_id": "evt_7cfba268285450a9c78c974569e7c722",
"message": [{
"name": "salmondx",
"isTest": true,
"formatted_amount": "$31.00",
"amount": 31,
"message": "This is a test donation for $31.00.",
"currency": "USD",
"from": "John"
}]
}
onHosting
Fires when other streamer hosts a user stream.
onHosting: function(onHostingCallback: function) callbackId: string
onHostingCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always 'host') |
message | array of objects | Array with details about host. |
for | string | Platform identifier of event (specifies from which platform did this event come from). Possible values are: |
message
array element object properties:
Property | Type | Description |
---|---|---|
name | string | Twitch name of the streamer who have hosted this stream |
viewers | number | Number of viewers from host stream |
isTest | bool | true if test event is fired from Streamlabs . |
onHosting
event example:
{
"type": "host",
"event_id": "evt_0b217929f95879e9dfa7647ef3be2b60",
"for": "twitch_account",
"message": [{
"name": "salmondx",
"isTest": true,
"viewers": 1320
}]
}
onBits
Fires when someone donate bits to a user on Twitch.
onBits: function(onBitsCallback: function) callbackId: string
onBitsCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always 'bits') |
message | array of objects | Array with details about bits donations. |
message
array element object properties:
Property | Type | Description |
---|---|---|
name | string | Twitch name of the bits donator |
amount | number | Bits donation amount |
message | string | Donator message |
isTest | bool | true if test event is fired from Streamlabs . |
onBits
event example:
{
"type": "bits",
"event_id": "evt_da94b1ba42fa3a23259b984ca97f20a6",
"message": [{
"name": "salmondx",
"isTest": true,
"amount": "500",
"message": "cheer500 this is a test bit alert"
}]
}
onRaid
Fires when streamer raids a user stream on Twitch.
onRaid: function(onRaidCallback: function) callbackId: string
onRaidCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always 'raid') |
message | array of objects | Array with details about raids. |
message
array element object properties:
Property | Type | Description |
---|---|---|
name | string | Twitch name of the streamer who have raided this stream |
raiders | number | Number of viewers in a raid |
isTest | bool | true if test event is fired from Streamlabs . |
onRaid
event example:
{
"type": "raid",
"event_id": "evt_5a1cee2adfecee58e13615ddef1bb8dc",
"message": [{
"name": "salmondx",
"isTest": true,
"raiders": 1245
}]
}
onMerch
Fires when someone buys stuff from Streamlabs Merch store.
onMerch: function(onMerchCallback: function) callbackId: string
onMerchCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always 'merch') |
message | array of objects | Array with details about merch buyers. |
message
array element object properties:
Property | Type | Description |
---|---|---|
from | string | Display name from merch page on Streamlabs |
name | string | Twitch username of a buyer if he was logged in to Streamlabs on merch page |
message | string | Message from buyer on merch page |
product | string | Name of a bought product |
isTest | bool | true if test event is fired from Streamlabs . |
onMerch
event example:
{
"type": "merch",
"event_id": "evt_3e837c869a7d52ea8010a588ca8c5a6f",
"message": [{
"name": "salmondx",
"isTest": true,
"message": "This is a test merch.",
"from": "John",
"product": "T-shirt"
}]
}
onSuperchat
Fires when YouTube
superchat event occurs (analog of Twitch Bits, donations that are integrated to YouTube ecosystem).
onSuperchat: function(onSuperchat: function) callbackId: string
onSuperchat
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always superchat ) |
message | array of objects | Array with superchat messages details |
message
array element object properties:
Property | Type | Description |
---|---|---|
channelId | string | YouTube channel ID |
channelUrl | string | YouTube channel URL |
name | string | Donator username |
comment | string | Donator superchat message content |
amount | string | Donation amount |
currency | string | Currency code |
displayString | string | Formatted amount with currency code |
messageType | number | Superchat message tier. |
createdAt | date | Event created at date |
onSuperchat
event example:
{
"event_id": "evt_71b8438593d9c1ca4e50b96ea16ebb11",
"type": "superchat",
"message": [
{
"id": "LCC.Cg8KDQoLWmpUemdzdU1vM2sSHAoaQ05PcW01UzJ3dElDRll2WkhBb2RLd0lBOWc0.028141892513880107",
"channelId": "UCec4hVEu3ZXE8qlRtUeK0DA",
"channelUrl": "http://www.youtube.com/channel/UCec4hVEu3ZXE8qlRtUeK0DA",
"name": "Kappa Lord",
"comment": "love the stream",
"amount": "2000000",
"currency": "USD",
"displayString": "$2.00",
"messageType": 2,
"createdAt": "2017-08-22 00:51:57",
}
]
}
onStreamlabels
Fires when streamlabels get updated.
onStreamlabels: function(onStreamlabelsCallback: function) callbackId: string
onStreamlabelsCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always '"streamlabels"') |
message | array of objects | Array with details about streamlabels |
onStreamlabels
event example:
message.hash marks the uniqueness of the message.
Events from platforms (mixer, youtube, mixer) contains platform name in event name (e.g. 90day_top_facebook_star_senders
). If event is without a platform it means that its for twitch.
Multiplatform events are visible only if user have several platforms connected to his account (e.g. if user have twitch and mixer connected to streamlabs account, only twitch and mixer events will be visible).
{
"hash": "eb1e2c4b4720ccb1bf708f6b3a67ab18",
"data": {
"donation_goal": "",
"most_recent_donator": "asdasd: $1,040.00",
"session_most_recent_donator": "asdasd: $1,040.00",
"session_donators": "asdasd: $1,040.00, salmondx: $3.00",
"total_donation_amount": "$1,275.00",
"monthly_donation_amount": "$1,040.00",
"weekly_donation_amount": "$0.00",
"30day_donation_amount": "$1,043.00",
"session_donation_amount": "$1,043.00",
"all_time_top_donator": "asdasd: $1,040.00",
"monthly_top_donator": "asdasd: $1,040.00",
"weekly_top_donator": "",
"30day_top_donator": "asdasd: $1,040.00",
"session_top_donator": "asdasd: $1,040.00",
"all_time_top_donators": "asdasd: $1,040.00, salmondx: $235.00",
"monthly_top_donators": "asdasd: $1,040.00",
"weekly_top_donators": "",
"30day_top_donators": "asdasd: $1,040.00, salmondx: $3.00",
"session_top_donators": "asdasd: $1,040.00, salmondx: $3.00",
"all_time_top_donations": "asdasd: $1,040.00, salmondx: $13.00, salmondx: $12.00, salmondx: $12.00, salmondx: $12.00, salmondx: $12.00, salmondx: $12.00, salmondx: $12.00, salmondx: $12.00, salmondx: $12.00",
"monthly_top_donations": "asdasd: $1,040.00",
"weekly_top_donations": "",
"30day_top_donations": "asdasd: $1,040.00, salmondx: $3.00",
"session_top_donations": "asdasd: $1,040.00, salmondx: $3.00",
"session_subscriber_count": "0",
"session_follower_count": "0",
"session_most_recent_follower": "",
"session_most_recent_subscriber": "",
"session_most_recent_resubscriber": "",
"session_subscribers": "",
"session_followers": "",
"most_recent_follower": "",
"most_recent_subscriber": "",
"most_recent_resubscriber": "",
"total_follower_count": "0",
"total_subscriber_count": "0",
"total_subscriber_score": "0",
"most_recent_cheerer": "",
"session_most_recent_cheerer": "",
"session_cheerers": "",
"total_cheer_amount": "",
"monthly_cheer_amount": "0",
"weekly_cheer_amount": "0",
"30day_cheer_amount": "0",
"session_cheer_amount": "0",
"all_time_top_cheerer": "",
"monthly_top_cheerer": "",
"weekly_top_cheerer": "",
"30day_top_cheerer": "",
"session_top_cheerer": "",
"all_time_top_cheerers": "",
"monthly_top_cheerers": "",
"weekly_top_cheerers": "",
"30day_top_cheerers": "",
"session_top_cheerers": "",
"all_time_top_cheers": "",
"monthly_top_cheers": "",
"30day_top_cheers": "",
"weekly_top_cheers": "",
"session_top_cheers": "",
"all_time_top_sub_gifters": "",
"monthly_top_sub_gifters": "",
"weekly_top_sub_gifters": "",
"30day_top_sub_gifters": "",
"session_top_sub_gifters": "",
"all_time_top_sub_gifter": "",
"monthly_top_sub_gifter": "",
"weekly_top_sub_gifter": "",
"30day_top_sub_gifter": "",
"session_top_sub_gifter": "",
"session_youtube_sponsor_count": "0",
"session_youtube_subscriber_count": "0",
"session_most_recent_youtube_subscriber": "",
"session_most_recent_youtube_sponsor": "",
"session_youtube_sponsors": "",
"session_youtube_subscribers": "",
"most_recent_youtube_subscriber": "",
"most_recent_youtube_sponsor": "",
"total_youtube_subscriber_count": "0",
"total_youtube_sponsor_count": "0",
"most_recent_youtube_superchatter": "",
"session_most_recent_youtube_superchatter": "",
"session_youtube_superchatters": "",
"total_youtube_superchat_amount": "$0.00",
"monthly_youtube_superchat_amount": "$0.00",
"weekly_youtube_superchat_amount": "$0.00",
"30day_youtube_superchat_amount": "$0.00",
"session_youtube_superchat_amount": "$0.00",
"all_time_top_youtube_superchatter": "",
"monthly_top_youtube_superchatter": "",
"weekly_top_youtube_superchatter": "",
"30day_top_youtube_superchatter": "",
"session_top_youtube_superchatter": "",
"all_time_top_youtube_superchatters": "",
"monthly_top_youtube_superchatters": "",
"weekly_top_youtube_superchatters": "",
"30day_top_youtube_superchatters": "",
"session_top_youtube_superchatters": "",
"all_time_top_youtube_superchats": "",
"monthly_top_youtube_superchats": "",
"30day_top_youtube_superchats": "",
"weekly_top_youtube_superchats": "",
"session_top_youtube_superchats": "",
"session_mixer_subscriber_count": "0",
"session_mixer_follower_count": "0",
"session_most_recent_mixer_follower": "",
"session_most_recent_mixer_subscriber": "",
"session_mixer_subscribers": "",
"session_mixer_followers": "",
"most_recent_mixer_follower": "",
"most_recent_mixer_subscriber": "",
"total_mixer_follower_count": "0",
"total_mixer_subscriber_count": "0",
"total_facebook_follower_count" : "",
"total_facebook_share_count" : "",
"total_facebook_like_count" : "",
"total_facebook_stars_count" : "",
"total_facebook_supporter_count" : "",
"session_facebook_follower_count" : "",
"session_most_recent_facebook_follower" : "",
"session_facebook_followers" : "",
"most_recent_facebook_follower" : "",
"session_facebook_share_count" : "",
"session_most_recent_facebook_share" : "",
"session_facebook_shares" : "",
"most_recent_facebook_share" : "",
"session_facebook_like_count" : "",
"session_most_recent_facebook_like" : "",
"session_facebook_likes" : "",
"most_recent_facebook_like" : "",
"session_facebook_supporter_count" : "",
"session_most_recent_facebook_supporter" : "",
"session_facebook_supporters" : "",
"most_recent_facebook_supporter" : "",
"90day_top_facebook_stars" : "",
"monthly_top_facebook_stars" : "",
"weekly_top_facebook_stars" : "",
"30day_top_facebook_stars" : "",
"session_top_facebook_stars" : "",
"90day_top_facebook_star_sender" : "",
"90day_top_facebook_star_senders" : ""
}
}
onUnderlyingStreamlabels
Fires when streamlabels get updated.
onUnderlyingStreamlabels: function(onUnderlyingStreamlabelsCallback: function) callbackId: string
onUnderlyingStreamlabelsCallback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
event_id | string | Unique identifier of event |
type | string | Event type (always '"streamlabels.underlying"') |
message | array of objects | Array with details about streamlabels |
onUnderlyingStreamlabels
event example:
message.hash marks the uniqueness of the message.
Events from platforms (mixer, youtube, mixer) contains platform name in event name (e.g. 90day_top_facebook_star_senders
). If event is without a platform it means that its for twitch.
Multiplatform events are visible only if user have several platforms connected to his account (e.g. if user have twitch and mixer connected to streamlabs account, only twitch and mixer events will be visible).
{
"hash": "eb1e2c4b4720ccb1bf708f6b3a67ab18",
"data": {
"donation_goal": "",
"most_recent_donator": {
"name": "asdasd",
"amount": "$1,040.00",
"message": "asdasdasd"
},
"session_most_recent_donator": {
"name": "asdasd",
"amount": "$1,040.00",
"message": "asdasdasd"
},
"session_donators": [
{
"name": "asdasd",
"amount": "$1,040.00",
"message": "asdasdasd"
},
{
"name": "salmondx",
"amount": "$3.00",
"message": "asdasdasdads"
}
],
"total_donation_amount": {
"amount": "$1,275.00"
},
"monthly_donation_amount": {
"amount": "$1,040.00"
},
"weekly_donation_amount": {
"amount": "$0.00"
},
"30day_donation_amount": {
"amount": "$1,043.00"
},
"session_donation_amount": {
"amount": "$1,043.00"
},
"all_time_top_donator": {
"name": "asdasd",
"amount": "$1,040.00"
},
"monthly_top_donator": {
"name": "asdasd",
"amount": "$1,040.00"
},
"weekly_top_donator": "",
"30day_top_donator": {
"name": "asdasd",
"amount": "$1,040.00"
},
"session_top_donator": {
"name": "asdasd",
"amount": "$1,040.00"
},
"all_time_top_donators": {
"name": "salmondx",
"amount": "$235.00"
},
"monthly_top_donators": {
"name": "asdasd",
"amount": "$1,040.00"
},
"weekly_top_donators": "",
"30day_top_donators": {
"name": "salmondx",
"amount": "$3.00"
},
"session_top_donators": {
"name": "salmondx",
"amount": "$3.00"
},
"all_time_top_donations": [
{
"name": "asdasd",
"amount": "$1,040.00",
"message": "asdasdasd"
},
{
"name": "salmondx",
"amount": "$13.00",
"message": "13123"
},
{
"name": "salmondx",
"amount": "$12.00",
"message": "13212313213"
},
{
"name": "salmondx",
"amount": "$12.00",
"message": "13212313213"
},
{
"name": "salmondx",
"amount": "$12.00",
"message": "13212313213"
},
{
"name": "salmondx",
"amount": "$12.00",
"message": "123123132"
},
{
"name": "salmondx",
"amount": "$12.00",
"message": "123123132"
},
{
"name": "salmondx",
"amount": "$12.00",
"message": "123123132"
},
{
"name": "salmondx",
"amount": "$12.00",
"message": "123123132"
},
{
"name": "salmondx",
"amount": "$12.00",
"message": "12312312313"
}
],
"monthly_top_donations": [
{
"name": "asdasd",
"amount": "$1,040.00",
"message": "asdasdasd"
}
],
"weekly_top_donations": [],
"30day_top_donations": [
{
"name": "asdasd",
"amount": "$1,040.00",
"message": "asdasdasd"
},
{
"name": "salmondx",
"amount": "$3.00",
"message": "asdasdasdads"
}
],
"session_top_donations": [
{
"name": "asdasd",
"amount": "$1,040.00",
"message": "asdasdasd"
},
{
"name": "salmondx",
"amount": "$3.00",
"message": "asdasdasdads"
}
],
"session_subscriber_count": "",
"session_follower_count": {
"count": "0"
},
"session_most_recent_follower": "",
"session_most_recent_subscriber": "",
"session_most_recent_resubscriber": "",
"session_subscribers": [],
"session_followers": [],
"most_recent_follower": "",
"most_recent_subscriber": "",
"most_recent_resubscriber": "",
"total_follower_count": {
"count": "0"
},
"total_subscriber_count": {
"count": "0"
},
"total_subscriber_score": {
"count": "0"
},
"most_recent_cheerer": "",
"session_most_recent_cheerer": "",
"session_cheerers": [],
"total_cheer_amount": {
"amount": null
},
"monthly_cheer_amount": {
"amount": 0
},
"weekly_cheer_amount": {
"amount": 0
},
"30day_cheer_amount": {
"amount": 0
},
"session_cheer_amount": {
"amount": 0
},
"all_time_top_cheerer": "",
"monthly_top_cheerer": "",
"weekly_top_cheerer": "",
"30day_top_cheerer": "",
"session_top_cheerer": "",
"all_time_top_cheerers": [],
"monthly_top_cheerers": "",
"weekly_top_cheerers": "",
"30day_top_cheerers": "",
"session_top_cheerers": "",
"all_time_top_cheers": [],
"monthly_top_cheers": [],
"30day_top_cheers": [],
"weekly_top_cheers": [],
"session_top_cheers": [],
"all_time_top_sub_gifters": [],
"monthly_top_sub_gifters": [],
"weekly_top_sub_gifters": [],
"30day_top_sub_gifters": [],
"session_top_sub_gifters": [],
"all_time_top_sub_gifter": [],
"monthly_top_sub_gifter": [],
"weekly_top_sub_gifter": [],
"30day_top_sub_gifter": [],
"session_top_sub_gifter": [],
"session_youtube_sponsor_count": {
"count": "0"
},
"session_youtube_subscriber_count": {
"count": "0"
},
"session_most_recent_youtube_subscriber": "",
"session_most_recent_youtube_sponsor": "",
"session_youtube_sponsors": "",
"session_youtube_subscribers": "",
"most_recent_youtube_subscriber": "",
"most_recent_youtube_sponsor": "",
"total_youtube_subscriber_count": {
"count": 0
},
"total_youtube_sponsor_count": {
"count": 0
},
"most_recent_youtube_superchatter": "",
"session_most_recent_youtube_superchatter": "",
"session_youtube_superchatters": [],
"total_youtube_superchat_amount": {
"amount": "$0.00"
},
"monthly_youtube_superchat_amount": {
"amount": "$0.00"
},
"weekly_youtube_superchat_amount": {
"amount": "$0.00"
},
"30day_youtube_superchat_amount": {
"amount": "$0.00"
},
"session_youtube_superchat_amount": {
"amount": "$0.00"
},
"all_time_top_youtube_superchatter": null,
"monthly_top_youtube_superchatter": "",
"weekly_top_youtube_superchatter": "",
"30day_top_youtube_superchatter": "",
"session_top_youtube_superchatter": "",
"all_time_top_youtube_superchatters": [],
"monthly_top_youtube_superchatters": "",
"weekly_top_youtube_superchatters": "",
"30day_top_youtube_superchatters": "",
"session_top_youtube_superchatters": "",
"all_time_top_youtube_superchats": [],
"monthly_top_youtube_superchats": [],
"30day_top_youtube_superchats": [],
"weekly_top_youtube_superchats": [],
"session_top_youtube_superchats": [],
"session_mixer_subscriber_count": {
"count": "0"
},
"session_mixer_follower_count": {
"count": "0"
},
"session_most_recent_mixer_follower": "",
"session_most_recent_mixer_subscriber": "",
"session_mixer_subscribers": [],
"session_mixer_followers": [],
"most_recent_mixer_follower": "",
"most_recent_mixer_subscriber": "",
"total_mixer_follower_count": {
"count": 0
},
"total_mixer_subscriber_count": {
"count": 0
}
}
}
onMessage
Fired for this user when you call a postMessage
method. This is a mechanism of real-time message passing between multiple pages of your application.
onMessage: function(callback: function) callbackId: string
callback
is a function with one argument, an object with these properties
Property | Type | Description |
---|---|---|
type | string | Type of event. Same as you send via postMessage |
data | object | Object from postMessage |
postMessage
This method is used to send real-time messages to your other pages (e.g. settings page, admin page, etc). You can send only 30 requests per minute
using this method.
postMessage: function(type: string, data: Object)
For example, if you have an application that consists of 2 pages and you want to pass the event to the page 2:
// page 1
// Skip streamlabs init step here
streamlabs.postMessage('myCustomType', {param1: '1', param2: '2'});
// page 2
streamlabs.onMessage(function(event) {
if (event.type === 'myCustomType') {
const param1 = event.data.param1;
const param2 = event.data.param2;
}
});
onChatMessage
DEPRECATED
Fired when someone types a message in this user's Twitch chat.
onChatMessage: function(onChatMessageCallback: function) callbackId: string
onChatMessageCallback
is a function with one argument, an object with these properties:
Property | Type | Description |
---|---|---|
from | string | Sender's twitch username |
body | string | Text body of the message |
platform | string | Platform name of this message event. Currently only twitch chat is supported. |
subscriber | bool |
|
to | string | Name of the room ( |
tags | array | Tags for this message event |
onChatEvent
DEPRECATED
Fired for ALL events which occurres in Twitch chat (NOTICE
, JOIN
, etc). Use only if you know how twitch IRC chat works.
You should use this type of event if you want to detect special IRC events, e.g. room joins (works only below 1000 viewers in chat), notice events from twitch server, etc. Take a raw
property from this event and parse it by your own.
onChatEvent: function(onChatEventCallback: function) callbackId: string
onChatEventCallback
is a function with one argument, an object with these properties:
Property | Type | Description |
---|---|---|
raw | string | Raw representation of twitch IRC event. |
command | string | Parsed twitch IRC command (e.g. PRIVMSG ) |
crlf | string | CRLF message content of twitch IRC chat event |
params | array | Parsed params from IRC event (e.g. name of the room). |
prefix | string | Prefix of this IRC event |
tags | array | Tags for this message event |
sendChatMessage
DEPRECATED
Send message to this user's Twitch chat. Works only if you initialized a Twitch Chat with twitch.initTwitchChat method.
sendChatMessage: function(message: string)
For example:
const streamlabs = window.Streamlabs;
streamlabs.init({ twitchChat: true, twitchAuth: { nick: 'test', token: '1312' }})
.then(_ => {
streamlabs.sendChatMessage('hello chat');
});
sendChatRaw
DEPRECATED
Send raw IRC command to this user's Twitch chat. Works only if you initialized a Twitch Chat with twitch.initTwitchChat method. Use this only if you know how Twitch IRC chat works.
sendChatRaw: function(message: string)
clear
Use this method to remove callback from triggering. You should call this method to clear callbacks state and to prevent listeners leak.
For example, you should use this method if you have dynamic views. You should clear listeners in your framework lifecycle methods (e.g. in Vue.js
call this method in beforeDestroy
method, in React
call this method in componentWillUnmount
lifecycle method).
clear: function(callbackId: string)
Example for Vue.js
:
<template>
....
</template>
<script>
export default {
data() {
return {
followListener: null,
};
},
mounted() {
// we have initialized SDK in main component, e.g.
this.followListener = window.Streamlabs.onFollow(event => {
console.log(event);
});
},
beforeDestroy() {
// we should clear all listeners before this component will be destroyed to preven leaks
window.Streamlabs.clear(this.followListener);
}
}
</script>
userSettings
You can use userSettings
API to store up to 50 settings for each user of your application. The max length of the key is 100 characters and the max length of the value is chunk of data that is less than 64k. userSettings
is a key-value
storage system and via this API you can store user preferences: e.g. custom colors, sound options, links, etc.
Using userSettings
API you can also store user assets such as audio and images in Streamlabs CDN.
userSettings.get
Get previously stored setting by key for current user.
get: function(key: string) Promise
Example:
window.Streamlabs.userSettings.get('my-custom-key')
.then(valueOrNull => {
console.log(valueOrNull);
});
userSettings.getAll
Get all stored settings for current user
getAll: function() Promise
Example:
window.Streamlabs.userSettings.getAll()
.then(settings => {
console.log(settings);
});
userSettings.set
Store string value by key for current user.
set: function(key: string, value: mixed) Promise
Example:
//store value as a string
window.Streamlabs.userSettings.set('my-custom-key', 'my-custom-key')
.then( _ => {
console.log('value saved!');
});
//store value as an json object, NOTICE, there is no need to use JSON.stringify
window.Streamlabs.userSettings.set('my-custom-color', {'color' : 'red'})
.then( _ => {
console.log('value saved!');
});
userSettings.delete
Delete previously store setting by key for current user.
delete: function(key: string) Promise
Example:
window.Streamlabs.userSettings.delete('my-custom-key')
.then(valueOrNull => {
console.log('key deleted!');
});
userSettings.deleteAll
Delete all stored settings for current user.
deleteAll: function() Promise
Example:
window.Streamlabs.userSettings.deleteAll()
.then(result => {
console.log(result);
});
userSettings.addAssets
Upload assets (images, audios) to Streamlabs CDN for current user. Maximum size of an item - 20 Mb
. Objects with the same name
properties will be replaced.
Supported file extensions:
Type | Extensions |
---|---|
image | png, jpeg, jpg, bmp, gif |
audio | wav, ogg, oga, spx, aiff, aif, mp3, mpga |
video | webm, mpeg, avi |
addAssets: function (assets: array) Promise
Where assets
is the array of objects:
Property | Type | Description |
---|---|---|
name | string | Name for provided asset. It is not the same as the For example, you can store user profile icon with name |
file | File that will be stored in Streamlabs CDN |
Example:
// html
...
<input type="file" id="input">
...
// js
const fileList = document.getElementById('input').files;
// FileList (https://developer.mozilla.org/en-US/docs/Web/API/FileList) is returned, we should convert it to JS array using ES6
const files = [...fileList];
// take first file and store it with name `user-icon`
const toCDN = { name: 'user-icon', file: files[0] };
// don't forget to pass an array even if you have a single file
streamlabs.userSettings.addAssets([ toCDN ])
.then(result => {
console.log('files stored! user icon url: ' + result['user-icon']);
});
userSettings.removeAssets
Remove assets from Streamlabs CDN.
removeAssets: function (names: array) Promise
Where names
is a string
array that contains names of stored assets.
streamlabs.userSettings.removeAssets(['user-icon'])
.then(_ => {
console.log('removed');
});
userSettings.getAssets
Retrieve previously stored assets.
getAssets: function() Promise
getAssets
returns a Promise that resolves with the js object where properties
are names of previously stored assets and their values
are url
with assets location.
For example, if we previously store image with name user-icon
, then response object will look like:
{
"user-icon": "https://cdn.streamlabs.com/path/to/image"
}
Assets are available ininit
PromiseAll user assets are loaded during
init
in a same format and availbe as other params (such assettings
,profiles
, etc). Details in init section
Example:
// previosly we stored an object with name `user-icon`
streamlabs.userSettings.addAssets([{ name: 'user-icon', file: file }]);
// getAssets example
streamlabs.userSettings.getAssets()
.then(response => {
console.log('Here is the user profile icon URL: ' + response['user-icon']);
});
streamlabels.getInitialData
Get streamlabels initial data
getInitialData: function() Promise
Example:
window.Streamlabs.streamlabels.getInitialData()
.then(data => {
console.log(data);
});
streamlabels.getUnderlyingInitialData
Get streamlabels underlying initial data.
getUnderlyingInitialData: function() Promise
Example:
window.Streamlabs.streamlabels.getUnderlyingInitialData()
.then(data => {
console.log(data);
});
twitch.connectTwitchChatByName
Connect twitch chat by chatroom name. It is useful when you need to test your app in a chat-heavy environment.
Example:
window.Streamlabs.twitch.connectTwitchChatByName("chatroom name");
twitch.initTwitchChat
Authorize with provided twitch oauth token to be able to write to twitch chat. Without authorization, sendChatMessage
and sendChatRaw
methods will not work.
Do not expose your OAuth token by hard-coding it in your source code or by returning it from your server. This function should only be used with end-user tokens OAuth tokens your app has acquired via the Streamlabs Desktop Authorization Module.
initTwitchChat: function (twitchAuth: object)
Where twitchAuth is an object with these fields:
Property | Type | Description |
---|---|---|
nick | string | Nickname of messages owner. Use username of twitch token owner. |
token | string | OAuth token for twitch IRC chat. Do not hardcode this token in code. We will decline your app submission if we will see a client tokens. |
Example:
window.Streamlabs.twitch.initTwitchChat({ nick: 'test_user', token: 'oauth:asdasd' });
alerts.createAlerts
Required Permissions: platform.create-alerts
Send a customized alert to the streamer
createAlerts: function(options: Object) Promise
options
is an Object with the following properties.
Property | Type | Description |
---|---|---|
type | string | This parameter determines which alert box this alert will show up in, and thus should be one of the following: follow, subscription, donation, or host. |
image_href | string | The href pointing to an image resource to play when this alert shows. If an empty string is supplied, no image will be displayed. |
sound_href | string | The href pointing to a sound resource to play when this alert shows. If an empty string is supplied, no sound will be played. |
message | string | The message to show with this alert. If not supplied, no message will be shown. Surround special tokens with s, for example: This is my special* alert! |
user_message | string | Acting as the second heading, this shows below message, |
duration | string | How many seconds this alert should be displayed. Value should be in milliseconds.Ex: 1000 for 1 second. |
special_text_color | string | The color to use for special tokens. Must be a valid CSS color string. |
Example:
window.Streamlabs.alerts.createAlerts(
{
message:'Really like your stream',
user_message: 'What is the meaning of life?'
}
);
alerts.mute
Required Permissions: platform.mute-alerts
Mute user's alert
window.Streamlabs.alerts.mute().then(
result=>console.log(result)
);
alerts.unmute
Required Permissions: platform.mute-alerts
Unmute user's alert
window.Streamlabs.alerts.unmute().then(
result=>console.log(result)
);
alerts.repeat
Required Permissions: platform.create-alerts
Repeat an alert
Example:
params.data
is the same as message[0]
in subscription event
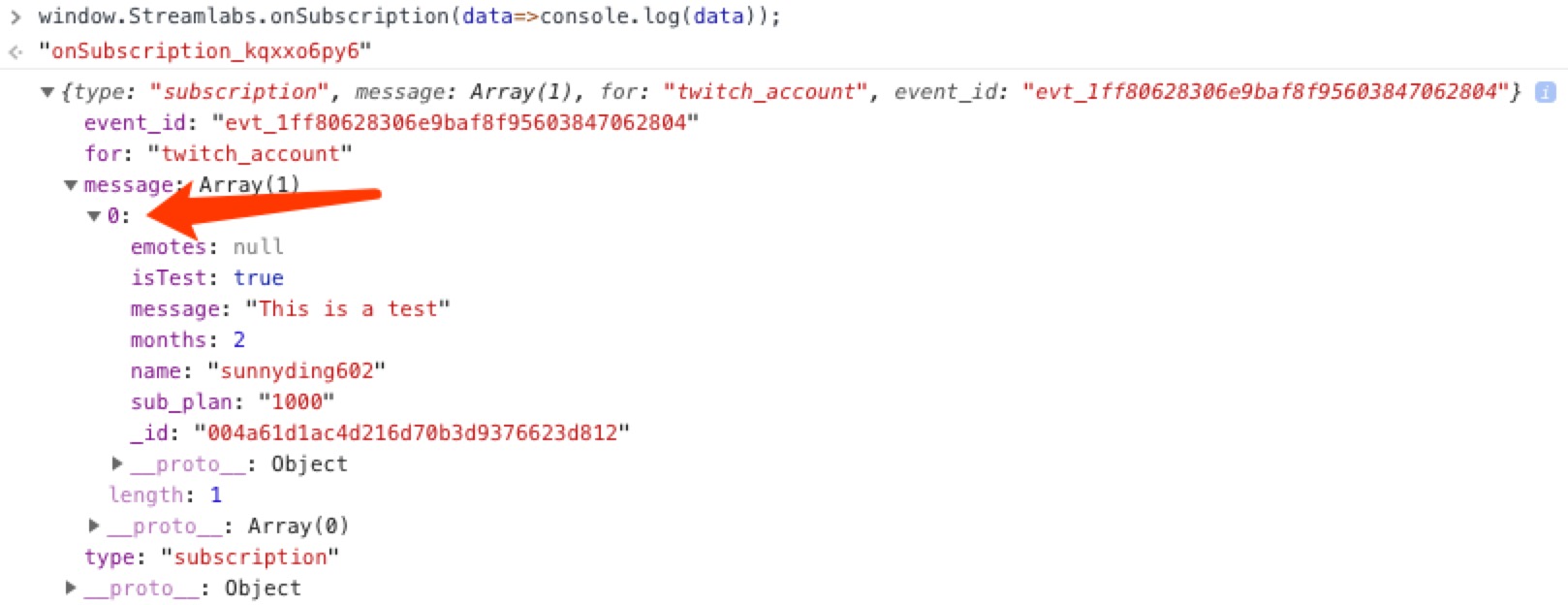
let params = {};
params.type = 'subscription';
params.data = {emotes: null,
isTest: true,
message: "This is a test",
months: 2,
name: "sunnyding602",
sub_plan: "1000",
_id: "IamAId"};
window.Streamlabs.alerts.repeat(params).then(
result=>console.log(result)
);
Updated about 1 month ago