Platform API
Overview
The Platform API is your primary API for interacting with the Streamlabs platform, as well as handling settings and communication for the various pieces of your app. To get access to this API, you should include our Platform API scrip in your HTML via a script tag.
This file is located at the URL:
https://cdn.streamlabs.com/slobs-platform/lib/streamlabs-platform.min.js
This script should be included at runtime via <script>
tag. It should not be compiled into your application bundle. This is the only script your application will be allowed to load at runtime.
1. Platform API script
To get access to our API, receive events, and get user profile data, you should include our Platform API script in your application.
Once the script is loaded, the API is available via a global object injected into window
and you can access it like in this example:
const streamlabs = window.Streamlabs;
streamlabs.init({ receiveEvents: true })
.then(data => {
console.log('Hello user: ' + data.profiles.twitch.display_name);
});
streamlabs.onDonation(event => {
console.log('donation received!');
});
You can use whatever JS framework that you want with this API. Just remember to initialize it once in the bootstrap section of your application. Then you can access this initialized global object in every component through window.Streamlabs
, so no need to pass it via properties of your components.
You can subscribe several times on a single type of event, for example, in the code below we have two handlers for the onFollow
event in two different components:
// component 1
window.Streamlabs.onFollow(event => {
console.log('follow event handler in 1st component');
});
// component 2
window.Streamlabs.onFollow(event => {
console.log('follow event handler in 2nd component');
});
If your application has dynamic views and routing, don't forget to clear
handlers in your framework's lifecycle methods to prevent leaking listeners (e.g. beforeDestroy
for Vue
or in componentWillUnmount
for React
). Example:
const callbackId = window.Streamlabs.onRaid(event => console.log('raid'));
// call clear method to remove this handler from state
window.Streamlabs.clear(callbackId);
2. Application Global Settings
These global settings are accessible for every user of your application. Here you can store whatever parameters that you want, up to 20 parameters per application.
You can change this parameters at any time and they will be available after next application restart.
To setup global settings, Edit
your application on platform.streamlabs.com
and open the App Settings
tab:
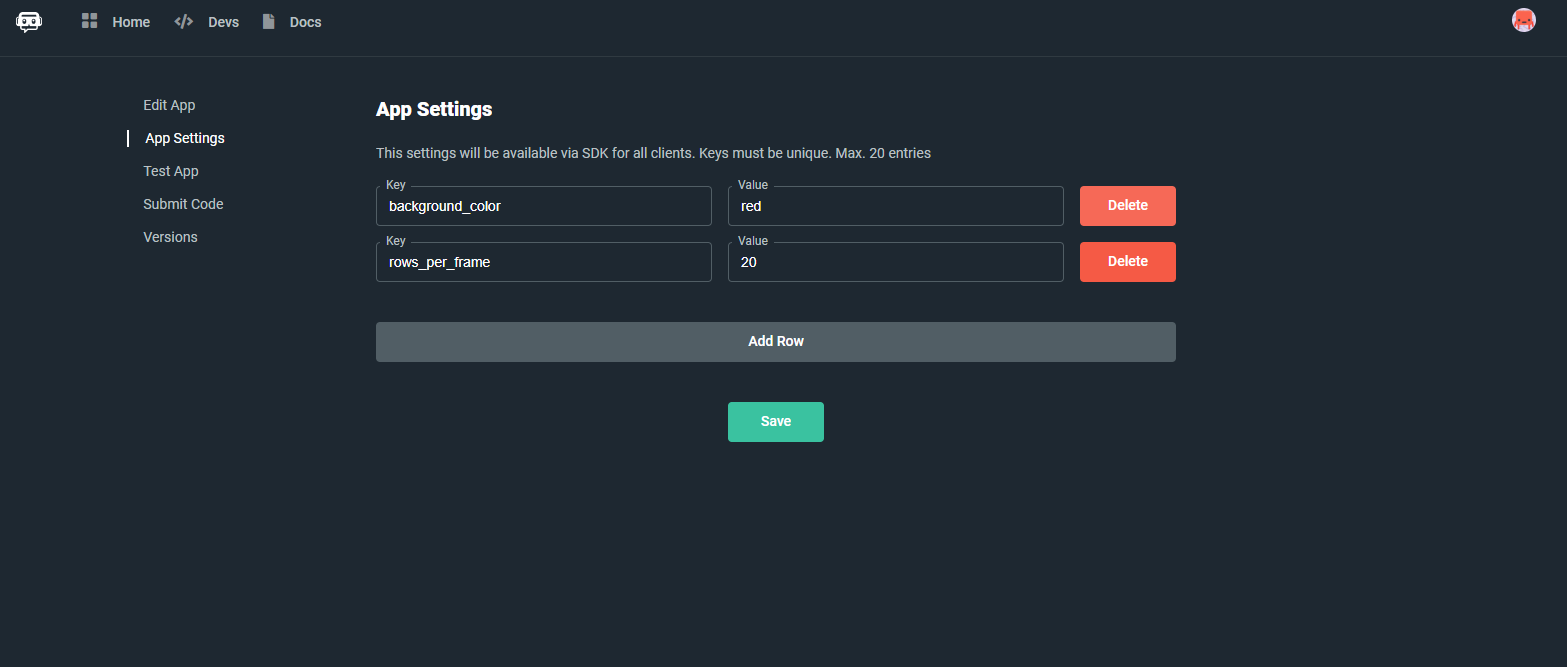
Application settings
These settings are available after API initialization in the settings
object property:
window.Streamlabs.init()
.then(data => {
// your settings are accessible via settings property
// with the same name as on platform.streamlabs.com page
const rows = data.settings.rows_per_frame;
const color = data.settings.background_color;
});
3. Real-time message passing
If you have multi-page application (e.g. main widget page and settings page for the streamer), you can pass events between them in real-time using our API.
For example, if you are building a music player application, on the settings page a streamer can select an audio track, and you can send the details of that track to your widget page to add it to the queue. This can be done using our message passing API:
// streamer settings page
/**
* audioTrack - regular JavaScript object
*/
addAudioTrack(audioTrack) {
window.Streamlabs.postMessage('newAudioTrack', audioTrack);
}
// widget page (running in browser source on stream)
// here we should add this audio track to queue
window.Streamlabs.onMessage(event => {
switch(event.type) {
case 'newAudioTrack':
this.addTrackToQueue(event.data);
break;
default:
}
});
4. Store user settings
You can save user settings for your application using our API. Currently, 50 entries per user are allowed. Common examples of these settings are custom colors, hide-unhide options, custom links, etc.
If you want to store more complex data, you should serialize it into a string using JSON or something similar.
Example:
//store a string "red" in key "color"
window.Streamlabs.userSettings.set('color', 'red')
.then(() => console.log('saved'));
window.Streamlabs.userSettings.get('color')
.then(color => console.log('Its red! ' + color));
//store an object {"color", "red"} in key "my_setting"
//NOTICE, no JSON.stringify or JSON.parse is needed.
window.Streamlabs.userSettings.set('my_setting', {'color': 'red'})
.then(() => console.log('saved'));
window.Streamlabs.userSettings.get('my_setting')
.then(mySetting => console.log('Its red! ' + mySetting.color));
5. Streamlabs Desktop native API
To interact with and control the host Streamlabs Desktop application, you should use the Streamlabs Desktop
API instead. You can find documentation about this API here: Streamlabs Desktop API
6. Store user assets (images and audio files) in Streamlabs CDN
With Streamlabs SDK it is possible to store different assets for your users. For example, you can store user custom images and audio files in Streamlabs CDN without your own backend. We support different file's extensions and each item can be up to 20 Mb in size.
Example:
// html
<input type="file" id="input" multiple>
<button onclick="upload()">Upload</button>
// js
window.streamlabs.init()
.then(data => {
console.log('SDK initialized');
});
function upload() {
const fileList = document.getElementById('input').files;
const toCDN = { name: 'user-icon', file: fileList[0] };
window.streamlabs.userSettings.addAssets([ toCDN ])
.then(data => {
console.log('image url:' + data['user-icon']);
});
}
Final Notes
This is a living API. This API is currently in a limited state and we plan on expanding it massively over the coming weeks and months. If there is any functionality that you wish was available, please let us know, and we can see if it is feasible to add it. We want this API to be driven by the people building and investing in the platform.
Please post in if you have suggestions or a question. We will figure it out together.
Updated over 2 years ago